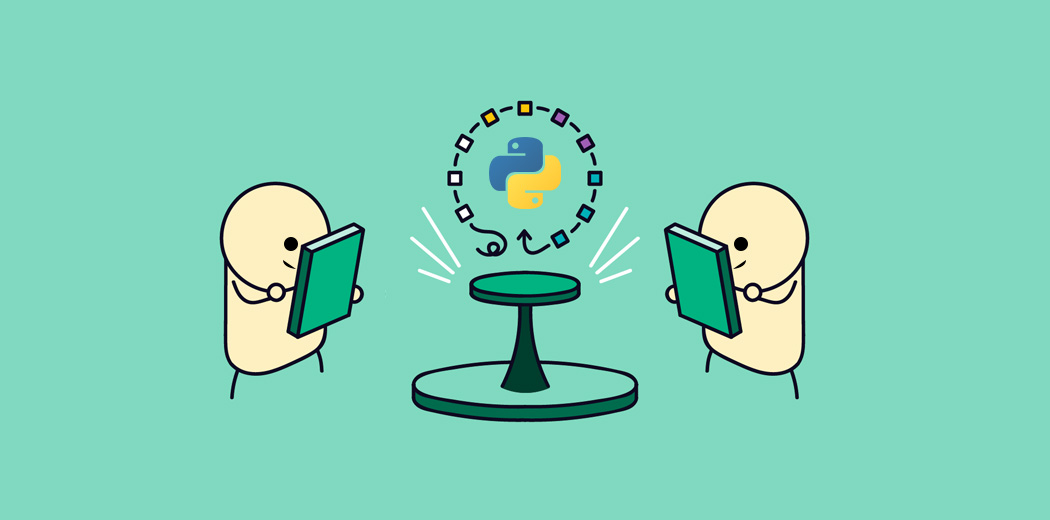
method()
The proper context for calling a regular method depends on whether the method returns a value or not.If the method returns a value, it is an expression and can be called from any context where an expression is valid.
If the method does not return a value, it is an action and can be called from any context where an action is valid.
1 <'
2 define HIGH 100;
3 define LOW 50;
4 struct memory_gen {
5 ! err_cnt : int;
6 ! war_cnt : int;
7 // Define a event for posedge of clock
8 event pos_clk is rise('top.clk')@sim;
9
10 // Define a method to check valid address
11 addr_valid(addr : byte ):bool is {
12 // Check if address is between HIGH and LOW value
13 // Wait for one cycle and drive command
14 if ((addr < LOW) || (addr > HIGH)) {
15 return FALSE;
16 } else {
17 return TRUE;
18 };
19 };
20
21 // This method prints the error and warning count
22 print_status() is {
23 outf("Number of Warnings : %d",war_cnt);
24 outf("Number of Errors : %d",err_cnt);
25 };
26
27 // We use this TCM to generate commands,drive them
28 // and call out methods
29 mem_txgen()@pos_clk is {
30 var addr : byte;
31 // Repeat (NO_COMMANDS)
32 // generate write/read commands
33 // Check address
34 if (addr_valid(addr)) {
35 // Drive to DUT
36 } else {
37 out ("address is not valid, skipping driver");
38 };
39 // End repeat
40 // Call the status method
41 print_status();
42 // Stop simulation
43 };
44 };
45 '>
Â
Â
1 <'
2 define HIGH 100;
3 define LOW 50;
4 define ERR_LIMIT 200;
5 struct memory_gen {
6 ! err_cnt : int;
7 ! war_cnt : int;
8 // Define a event for posedge of clock
9 event pos_clk is rise('top.clk')@sim;
10
11 // Define a method to check valid address
12 addr_valid(addr : byte ):bool is {
13 // Check if address is between HIGH and LOW value
14 // Wait for one cycle and drive command
15 if ((addr < LOW) || (addr > HIGH)) {
16 return FALSE;
17 } else {
18 return TRUE;
19 };
20 };
21
22 // This method prints the error and warning count
23 print_status():bool is {
24 outf("Number of Warnings : %d",war_cnt);
25 outf("Number of Errors : %d",err_cnt);
26 if (err_cnt >= ERR_LIMIT) {
27 return FALSE;
28 } else {
29 return TRUE;
30 };
31 };
32
33 // We use this TCM to generate commands,drive them
34 // and call out methods
35 mem_txgen()@pos_clk is {
36 var addr : byte;
37 // Repeat (NO_COMMANDS)
38 // generate write/read commands
39 // Check address
40 if (addr_valid(addr)) {
41 // Drive to DUT
42 } else {
43 out ("address is not valid, skipping driver");
44 };
45 // End repeat
46 // Call the status method, Since it returns a value
47 // Which is no use to us, we use 'compute'
48 compute print_status();
49 // Stop simulation
50 };
51 };
Â
Â
1 <'
2 define HIGH 100;
3 define LOW 50;
4 define ERR_LIMIT 200;
5 struct memory_gen {
6 ! err_cnt : int;
7 ! war_cnt : int;
8 // Define a event for posedge of clock
9 event pos_clk is rise('top.clk')@sim;
10
11 // Define a method to check valid address
12 // This method shows how to use result
13 addr_valid(addr : byte ):bool is {
14 // Check if address is between HIGH and LOW value
15 // Wait for one cycle and drive command
16 if ((addr < LOW) || (addr > HIGH)) {
17 result = FALSE;
18 } else {
19 result = TRUE;
20 };
21 };
22
23 // This method prints the error and warning count
24 // This method shows how to use return
25 print_status():bool is {
26 outf("Number of Warnings : %d",war_cnt);
27 outf("Number of Errors : %d",err_cnt);
28 if (err_cnt >= ERR_LIMIT) {
29 return FALSE;
30 } else {
31 return TRUE;
32 };
33 };
34
35 // This method shows how to use return without a value
36 print_parity(data:byte, addr:byte) is {
37 if (addr_valid(addr)) {
38 var parity : bit = data[7:7]^data[6:6]^data[5:5]^
39 data[4:4]^data[3:3]^data[2:2]^
40 data[1:1]^data[0:0];
41 outf ("Parity of data is %b\n", parity);
42 } else {
43 return;
44 // This will not be executed
45 outf ("Address is not valid");
46 };
47 };
48
49 // We use this TCM to generate commands,drive them
50 // and call out methods
51 mem_txgen()@pos_clk is {
52 var addr : byte;
53 // Repeat (NO_COMMANDS)
54 // generate write/read commands
55 // Check address
56 if (addr_valid(addr)) {
57 // Drive to DUT
58 } else {
59 out ("address is not valid, skipping driver");
60 };
61 // End repeat
62 // Call the status method, Since it returns a value
63 // Which is no use to us, we use 'compute'
64 compute print_status();
65 // Stop simulation
66 };
67 };
68 '>
Bạn Có Đam Mê Với Vi Mạch hay Nhúng - Bạn Muốn Trau Dồi Thêm Kĩ Năng
Mong Muốn Có Thêm Cơ Hội Trong Công Việc
Và Trở Thành Một Người Có Giá Trị Hơn
Mong Muốn Có Thêm Cơ Hội Trong Công Việc
Và Trở Thành Một Người Có Giá Trị Hơn